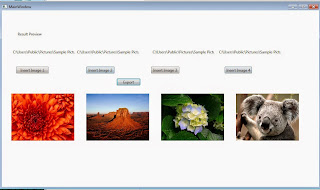
This is a Banner creator for one of our users since she doesnt know how to combine pictures.
The idea is make a Banner with 4 pictures, so she can easily add pictures and click export button to save it into one.
It was referenced from http://stackoverflow.com/questions/14661919/merge-png-images-into-single-image-in-wpf
Also as you can see, if we play with the template a bit and choose different combination of picture orders and also make a single radio seletion list, the combine method can be overload as many way as you want. (Vertical, Rectangle, etc) You only need specify how the image frames are drawn. Also if you enable drop on the controls, you can also get drop file directly however you may need go through all the control by name and assgn the files to them. There would be a lot potential on this. However the core functions are below.
Code:
MainWindow.xaml
MainWindow.xaml.cs
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows; using System.Windows.Controls; using System.Windows.Data; using System.Windows.Documents; using System.Windows.Input; using System.Windows.Media; using System.Windows.Media.Imaging; using System.Windows.Navigation; using System.Windows.Shapes; using Microsoft.Win32; using System.Drawing; using System.IO; namespace Draw { ////// Interaction logic for MainWindow.xaml /// public partial class MainWindow : Window { public MainWindow() { InitializeComponent(); } public void Button_Click(object sender, RoutedEventArgs e) { OpenFileDialog op = new OpenFileDialog(); op.Title = "Select a picture"; op.Filter = "All supported graphics|*.jpg;*.jpeg;*.png|" + "JPEG (*.jpg;*.jpeg)|*.jpg;*.jpeg|" + "Portable Network Graphic (*.png)|*.png"; if (op.ShowDialog() == true) { image1.Source = new BitmapImage(new Uri(op.FileName)); } lb1.Content = op.FileName; } private void Button_Click_1(object sender, RoutedEventArgs e) { OpenFileDialog op2 = new OpenFileDialog(); op2.Title = "Select a picture"; op2.Filter = "All supported graphics|*.jpg;*.jpeg;*.png|" + "JPEG (*.jpg;*.jpeg)|*.jpg;*.jpeg|" + "Portable Network Graphic (*.png)|*.png"; if (op2.ShowDialog() == true) { image2.Source = new BitmapImage(new Uri(op2.FileName)); } lb2.Content = op2.FileName; } private void Button_Click_2(object sender, RoutedEventArgs e) { OpenFileDialog op3 = new OpenFileDialog(); op3.Title = "Select a picture"; op3.Filter = "All supported graphics|*.jpg;*.jpeg;*.png|" + "JPEG (*.jpg;*.jpeg)|*.jpg;*.jpeg|" + "Portable Network Graphic (*.png)|*.png"; if (op3.ShowDialog() == true) { image3.Source = new BitmapImage(new Uri(op3.FileName)); } lb3.Content = op3.FileName; } private void Button_Click_3(object sender, RoutedEventArgs e) { OpenFileDialog op4 = new OpenFileDialog(); op4.Title = "Select a picture"; op4.Filter = "All supported graphics|*.jpg;*.jpeg;*.png|" + "JPEG (*.jpg;*.jpeg)|*.jpg;*.jpeg|" + "Portable Network Graphic (*.png)|*.png"; if (op4.ShowDialog() == true) { image4.Source = new BitmapImage(new Uri(op4.FileName)); } lb4.Content = op4.FileName; } public void COmbine(string filepath) { // Loads the images to tile (no need to specify PngBitmapDecoder, the correct decoder is automatically selected) BitmapFrame frame1 = BitmapDecoder.Create(new Uri(lb1.Content.ToString()), BitmapCreateOptions.None, BitmapCacheOption.OnLoad).Frames.First(); BitmapFrame frame2 = BitmapDecoder.Create(new Uri(lb2.Content.ToString()), BitmapCreateOptions.None, BitmapCacheOption.OnLoad).Frames.First(); BitmapFrame frame3 = BitmapDecoder.Create(new Uri(lb3.Content.ToString()), BitmapCreateOptions.None, BitmapCacheOption.OnLoad).Frames.First(); BitmapFrame frame4 = BitmapDecoder.Create(new Uri(lb4.Content.ToString()), BitmapCreateOptions.None, BitmapCacheOption.OnLoad).Frames.First(); // Gets the size of the images (I assume each image has the same size) // Draws the images into a DrawingVisual component DrawingVisual drawingVisual = new DrawingVisual(); using (DrawingContext drawingContext = drawingVisual.RenderOpen()) { drawingContext.DrawImage(frame1, new Rect(0, 0, 237, 150)); drawingContext.DrawImage(frame2, new Rect(238, 0, 237, 150)); drawingContext.DrawImage(frame3, new Rect(476,0 , 237, 150)); drawingContext.DrawImage(frame4, new Rect(714, 0, 237, 150)); } // Converts the Visual (DrawingVisual) into a BitmapSource RenderTargetBitmap bmp = new RenderTargetBitmap(950, 150, 96, 96, PixelFormats.Pbgra32); bmp.Render(drawingVisual); // Creates a PngBitmapEncoder and adds the BitmapSource to the frames of the encoder PngBitmapEncoder encoder = new PngBitmapEncoder(); encoder.Frames.Add(BitmapFrame.Create(bmp)); // Saves the image into a file using the encoder using (Stream stream = File.Create(filepath)) encoder.Save(stream); } private void Button_Click_4(object sender, RoutedEventArgs e) { SaveFileDialog fp = new SaveFileDialog(); fp.Filter = "JPG|*.JPG"; if (fp.ShowDialog() == true) { COmbine(fp.FileName); } } } }
No comments:
Post a Comment